Next.js Server Action and Sendgrid
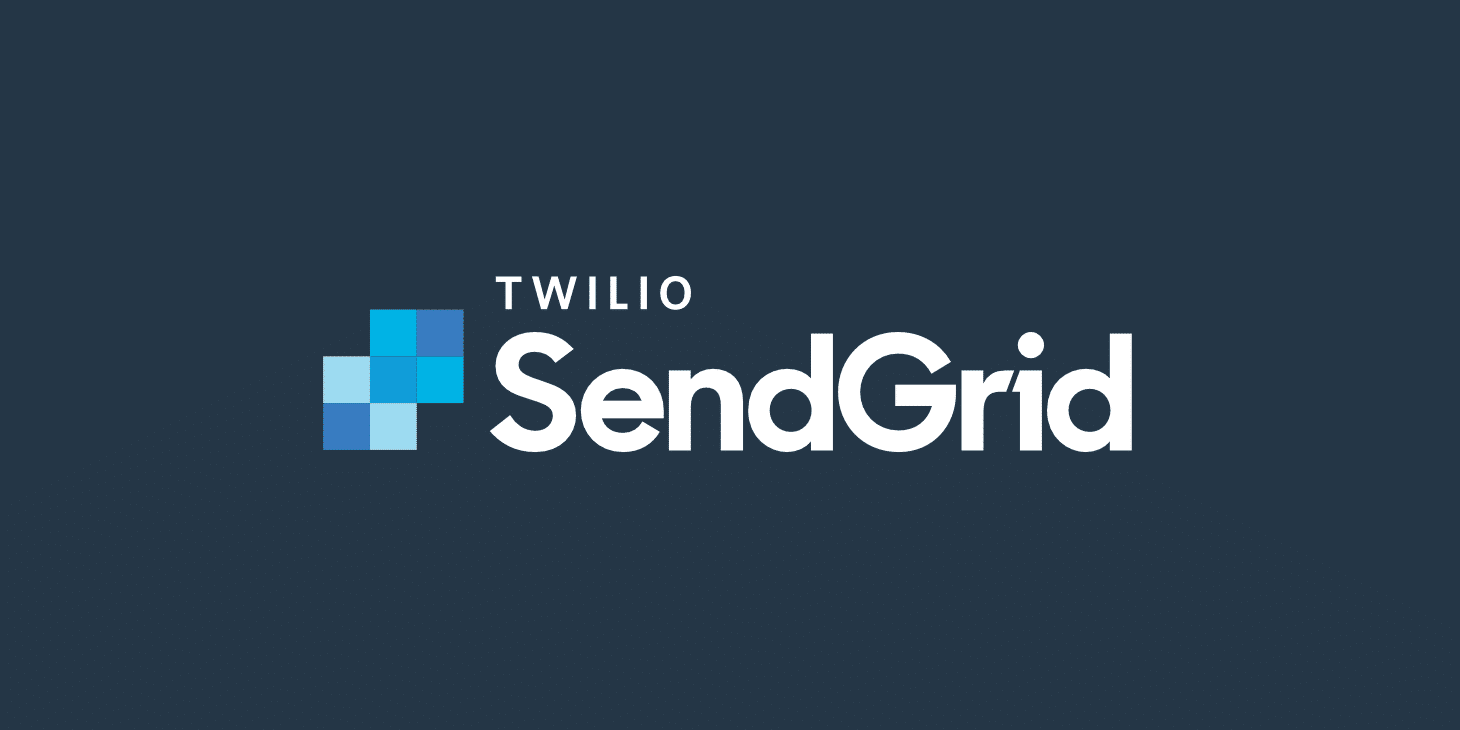
This is an old blog article that I’m updating today, 09/23/2024. The previous information was overkill. I was using a server action to pass data to a route handler—both were performing the same POST request, causing unnecessary duplication and network calls. Since writing the original article, I’ve gained a better understanding of React Server Components, Server Actions, and Route Handlers.
The purpose of this article was to demonstrate how to convert my contact form from the Page Router to the App Router. When the App Router was first introduced, I really struggled with this challenge.
My goal was to learn how to implement Next.js Server Actions while converting my contact page into a server component.
First, let’s discuss the concept of FormData. This isn’t difficult to grasp, and the code required is minimal. You pass FormData as a parameter to the function you create, which is then supplied to the action attribute of the form element in JSX.


If you console log the data returned to the function’s formData parameter, you’ll see your form input values returned. When the function executes, the formData parameter is populated with an object containing the values typed into your input fields.

Since we now have an object to work with, we can use methods, specifically get(), from the FormData interface. I extracted the values and placed them into another object, which I sent with a POST request.

Once you have the form input data, you can import SendGrid and supply it with your secret API key.


Next, we supply SendGrid with the data we want to submit from the form.

Here, I used JavaScript’s try-catch logic to ensure everything works as expected. On success, the form is submitted; on error, the form logs the error on the server side.

Here, I used JavaScript’s try-catch logic to ensure everything works as expected. On success, the form is submitted; on error, the form logs the error on the server side.



In the end, the code was very straightforward and minimal. My server action file barely exceeded 38 lines.

As for the HTML of the form, I haven’t included it here, as I’ll leave that up to your imagination. You can structure the HTML however you like for your form. I hope this article helps someone!